If you're using the Elements compiler for your iOS development, you can take advantage of a special additional behavior of the if #available
syntax in Elements' Swift implementation: in addition to making any code surrounded by the check execute conditionally at runtime, in Elements the check also implies a compile-time #if
check for the specified SDK.
What this means is that you can start using new APIs available only in the latest version of an SDK, but still compile your codebase against an older SDK, if needed. That's especially helpful during the beta period, as it allows you to play around with new APIs in, say, iOS 13 in your local builds and TestFlight deployment, while maintaining the ability to still ship updates to the app store based on iOS 12.2.
So for example, if you want to replace some images in your app with the excellent new SF Symbols APIs Apple introduced last week, you could write:
if #available(iOS 13.0) {
imageView.image = UIImage.systemImageNamed("mail") // use SF Symbols
} else {
imageView.image = UIImage.imageNamed("mail-icon") // use old icon in iOS 12
}
Then, simply select a different Target SDK version for your Release configuration vs. the Debug one. You can get your app ready for the fall in Debug, but if at any time you want to ship an interim update to your users before iOS 13 is out, just build Release and you'll be save to submit to the app store:
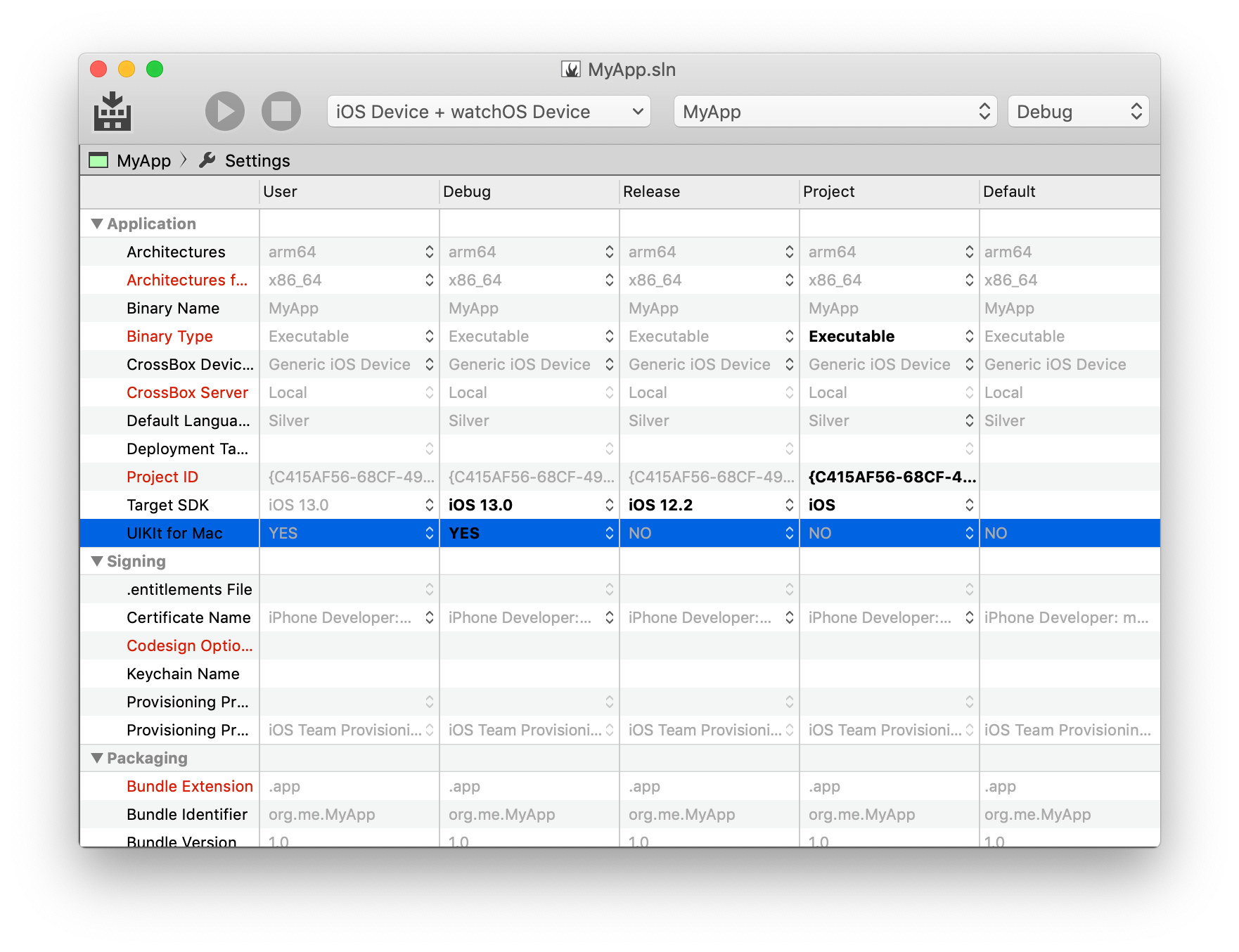
Of course the same functionality is also available in the other four Elements languages, Oxygene, C#, Java and Go, via the available()
System Function. The feature is available for both Cocoa and Android.
if available("iOS 13.0") then
imageView.image := UIImage.systemImageNamed("mail") // use SF Symbols
else
imageView.image := UIImage.imageNamed("mail-icon") // use old icon in iOS 12
Read more about Elements at elementscompiler.com.